USA
Configuring Webpack without Webpack
Webpack is a very powerful tool for module bundling in modern Frontend. It uses a paradigm where any kind of resource is a module: JavaScript, CSS, HTML, Images, Fonts, etc. The dark side of Webpack is its configuration API. It's not intuitive and takes a lot of time to master. Using Webpack is not easy for those who try it for the first time. There are stories when people spent days and weeks to configure a project infrastructure correctly.
What if there would be a way to encapsulate the complexity of Webpack? Is it possible to setup the whole infrastructure just with npm install command and spend 0 hrs for configuration? This is what Neutrino was invented for.
Neutrino is a CLI tool, which lets you build JavaScript applications (in browsers or NodeJS) using presets. Its goal is to make the process of initializing and building projects as simple as possible. Neutrino uses Webpack under the hood and webpack-chain library for configuration of presets and middlewares. Presets give you the necessary functionality for your project, such as language support, linting, testing, etc. So you will use all advantages of Webpack without even knowing Webpack.
This article describes how to quickstart with Neutrino v6, the latest version at the time of this writing.
React Project Preset
Let’s say we have a react-project folder, in which we want to initialize and start our application using React. We are going to display some simple text on the screen just to proof that everything works as expected. Also we would like to have tests in the future, so let’s setup them too.
Installation
Open a terminal in this folder and follow the next steps.
First, initialize the application manifest.
This will create a package.json file with default values. They are not so important for us right now.
Now we will install Neutrino plus its presets for React and Jest as development dependencies. Also we will install React and its complimentary packages.
react-project> npm install –save-dev neutrino neutrino-preset-react neutrino-preset-jest
Neutrino provides 3 standard commands: neutrino start, neutrino build, neutrino test. These 3 are for starting in the development mode, building for production, and testing, respectively. But we can’t call them directly because neutrino is not registered as a command in the system. That’s why we need to use NPM scripts.
In the package.json, add this section
“scripts”: {
“start”: “neutrino start –use neutrino-preset-react”,
“build”: “neutrino build –use neutrino-preset-react”,
“test”: “neutrino test –use neutrino-preset-react neutrino-preset-jest”
}
}
Development
Neutrino provides some conventions in the project file structure (which can be customized). Your source code should be located in the /src folder and your entry point should be named /src/index.*, where * is any supported extension that presets support, e.g. /src/index.js or /src/index.jsx. So let’s create this main file and edit it with:
/src/index.jsx
import { render } from ‘react-dom’;
function Header(){
return <h1>Application started</h1>;
}
render(<Header />, document.getElementById(‘root’));
Now you can run the command to build the application in the development mode and start Webpack DevServer with live reload. Run npm start command:
✔ Development server running on: http://localhost:5000
✔ Build completed
Open http://localhost:5000 in your browser, and you will see the page rendered. Any changes in source files will recompile only the changed modules and reload the page.
Building
Neutrino bundles the project into /build directory by default. Run npm run build command:
react-project> npm run build
✔ Building project completed
Hash: b26ff013b5a2d5f7b824
Version: webpack 2.6.1
Time: 9773ms
Asset Size Chunks Chunk Names
index.dfbad882ab3d86bfd747.js 181 kB index [emitted] index
polyfill.57dabda41992eba7552f.js 69.2 kB polyfill [emitted] polyfill
runtime.3d9f9d2453f192a2b10f.js 1.51 kB runtime [emitted] runtime
index.html 846 bytes [emitted]
You can either serve or deploy the contents of this /build directory as a static site.
Testing
For testing, you need to create files that end with .test.js in a /test directory. Let’s make a simple test for sanity. Create /test/index.test.js and edit it with the following code:
/test/index.test.js
it(‘should be sane’, () => {
expect(false).not.toBe(true);
});
});
Now call npm test command to run tests:
PASS test/index.test.js
simple
✓ should be sane (2ms)
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Snapshots: 0 total
Time: 0.972s
Ran all test suites.
To run tests against files from your source code, just import them using ES modules syntax.
Neutrino Jest preset also provides coverage reporting and many other customization options.
Presets and middlewares
The whole setup should take 5-10 mins. And you get:
- ES6 to ES5 compiler,
- ES modules with “tree shaking”,
- Dev Server with HMR for CSS
- JSX support
- development and production modes
- Webpack loaders for images, fonts, styles, and webworkers
- JavaScript polyfills for target browsers
- minification and source maps generation.
Many aspects of the presets may be overridden and customized.
We’ve discovered only small features of Neutrino ecosystem. You can also setup:
- linting,
- integration with code editor static code analyzers,
- test coverage,
- different frameworks presets,
- different Frontend languages support,
- more in the future.
Neutrino has a list of useful presets and middlewares in the core. All of them may be found on GitHub. But there are also many created by the community.
Conclusion
Using Neutrino gives a lot of new opportunities:
- Easy for anyone to start developing in Frontend. No matter if you are a newcomer or a switch from another language, you will get a maximum from the build tools.
- Speed. Using Webpack slows you down at the beginning, but gives you speed in the long term. And with Neutrino you are fast all the time.
- Project standards for teams and companies. If you want to create some corporate standard on how to setup a project infrastructure, it can be easily done with Neutrino. Custom presets can be created and shared between teams and projects. Any changes in the preset may be simply updated in projects using npm update command.
- CLI tool for custom frameworks. If you develop your own modern (React killer) Frontend framework, it is better to provide a CLI tool. With Neutrino it’s not a problem.
- Get Webpack updates for free. Very often Neutrino upgrades versions of Webpack, plugins, and loaders internally. So you don’t have to worry about migration from Webpack 2 to Webpack 4, resolve issues with Webpack DevServer, etc. The only thing you need is to be compatible with Neutrino configuration format.
Neutrino may remind you of creact-react-app, angular-cli or vue-cli. They play on the same field. But Neutrino is way more flexible. You may customize 3rd party presets and add your own ones. And in most cases it will not brake the upgrade process to new versions of Neutrino. It is universal. You may use it with React, Angular, VueJS, or any specific type of project.

Constantine is a Senior Frontend Developer and lecturer at many Frontend courses. He has a working knowledge in JavaScript, HTML, CSS, and much more. What is even more important, Constantine is ready to share his attainments.
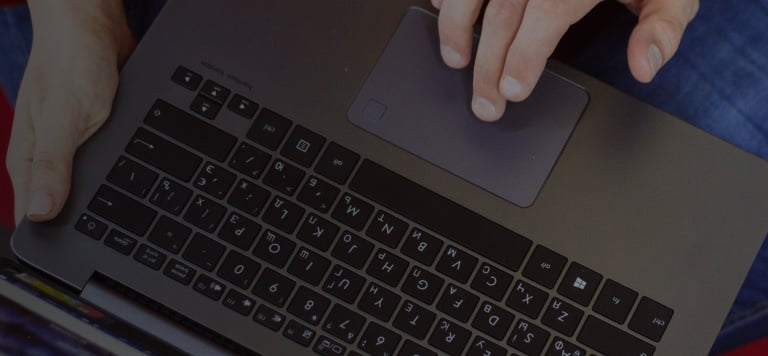
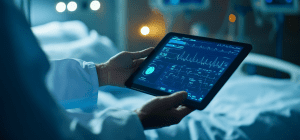
This in-depth guide on real-time patient monitoring platforms covers the main benefits for transplant centers, presents a list of key features for such platform...
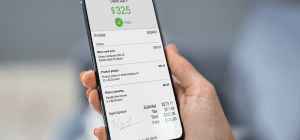
Every billing error isn’t just a technical issue — it’s a hit to your bottom line, a risk to your reputation, and a threat to patient trust. This guide breaks d...
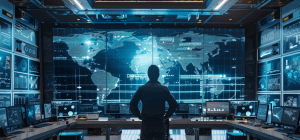
The role of the Chief Information Security Officer (CISO) has undergone a significant transformation in recent years. Today’s CISOs are not only responsible for...